Usage of this in React - Decoded
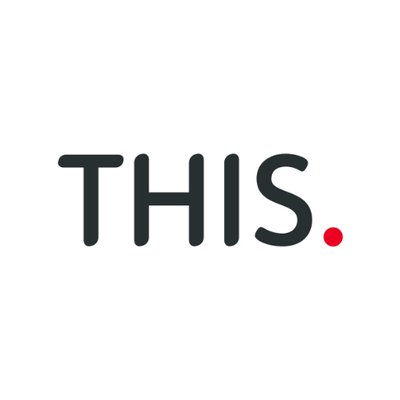
I am currently trying out small examples in react, when I came across many statements that explicitly re-declared the methods inside the Class Component as below:
constructor(props) {
super(props);
...
this.handleClick = this.handleClick.bind(this);
}
I wondered why. There are some rules when it comes to this binding in JavaScript.
- When a function is called in global scope, this refers to the global scope.
- When a function is called within a context object, the this reference will be bound to this object.
- When methods like .call(), .apply(), .bind() are used, this is bound to that object passed.
- Sometimes, this points to dynamic context depending on where, how and why the function is called. For example, when setTimeout or setInterval methods are used, they will clear out the contexts in the specified intervals of time. Hence, we should save the this to reuse them.
- When new is used to instantiate a new object like below, this points to that newly created object.
function Rectangle(l,b) {
this.l = l;
this.b = b;
}
Rectangle rect = new Rectangle(10,4);
That said, Arrow functions are completely different in the fact that they take the lexical this to perform the function. They take the scope of the enclosing object that calls this function.
this in arrow function always refers to the enclosing this
How this can be handled in React?
1. Binding in the constructor:
constructor(props) {
super(props);
...
this.handleClick = this.handleClick.bind(this);
}
handleClick() { }
render() => {
<button onClick={this.handleClick}>Click!</button>
}
2. Using Arrow functions:
render() => {
<button onClick={this.handleClick}>Click!</button>
}
handleClick = () => { }
3. Binding during the rendering:
constructor(props) {
super(props);
...
}
render() => {
<button onClick={this.handleClick.bind(this)}>Click!</button>
}
handleClick = () => { }